Lab 2 - Bluetooth:
The purpose of this lab was to establish communication between my computer and the Artemis board through the BLE bluetooth stack. I used Python inside a Jupyter notebook on the computer end and the Arduino programming language on the Artemis side.
Setup:
My setup ran smoothly, but ended up becoming moot. Due to an unexpected issue with Windows 11, I was unable to connect to the Artemis from my personal computer using Bluetooth. I instead used a lab computer to complete this lab, and plan to install a VM for future lab work. The lab computer was already fully set up with Arduino, Python, and Jupyter labs.
Tasks:
Below are screenshots of my Jupyter code/output and the Arduino Serial output. I also include my relevant Arduino code at the bottom.Task 1:
In my Arduino ECHO handler I prepend the string "Artemis says -> \"" and append "\"" to the string received from the Bluetooth command string. This string is returned and read on Jupyter.
Task 2:
My Arduino SEND_THREE_FLOATS handler gets all three float values from the command string and prints them comma separated to the Serial monitor.
Task 3:
My Jupyter notification handler stores continuously updated floats in the variable received_float and prints them using the callback function float_receiver.
Task 4:
The Arduino structs are sent over Bluetooth as a GATT characteristic, which is interpreted by Python as a bytearray. In the first case, the byte array represents a float and is directly unpacked that way by the receive_float() function. In the second case, the bytearray represents is an array of chars that corresponds to the float, and that char array is unpacked and later converted.
Serial Output

Jupyter Code/Output
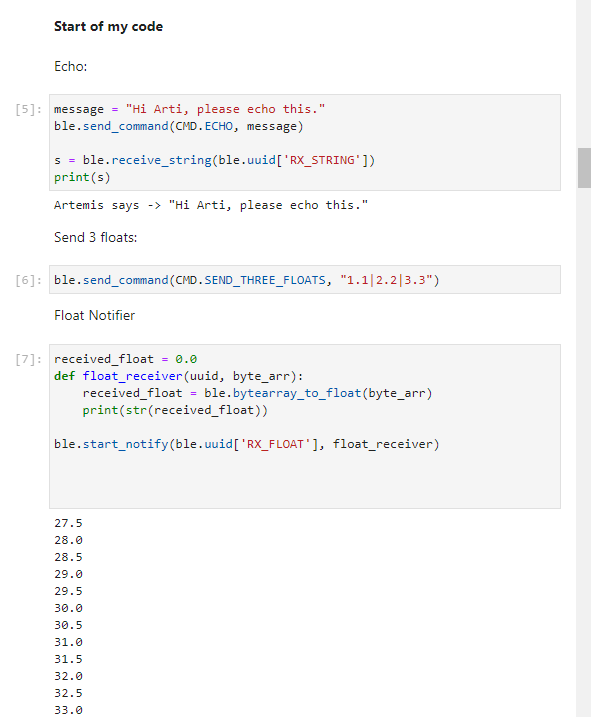
Arduino Code
/* * Extract three floats from the command string */ case SEND_THREE_FLOATS: float float_1, float_2, float_3; // Extract the next value from the command string as a float success = robot_cmd.get_next_value(float_1); if (!success) return; // Extract the next value from the command string as a float success = robot_cmd.get_next_value(float_2); if (!success) return; // Extract the next value from the command string as a float success = robot_cmd.get_next_value(float_3); if (!success) return; Serial.print("Received 3 Floats: "); Serial.print(float_1); Serial.print(", "); Serial.print(float_2); Serial.print(", "); Serial.print(float_3); Serial.print("\n"); break; /* * Add a prefix and postfix to the string value extracted from the command string */ case ECHO: char char_arr[MAX_MSG_SIZE]; // Extract the next value from the command string as a character array success = robot_cmd.get_next_value(char_arr); if (!success) return; tx_estring_value.clear(); tx_estring_value.append("Artemis says -> \""); tx_estring_value.append(char_arr); tx_estring_value.append("\""); tx_characteristic_string.writeValue(tx_estring_value.c_str()); break;